Getting started with Brightway25!
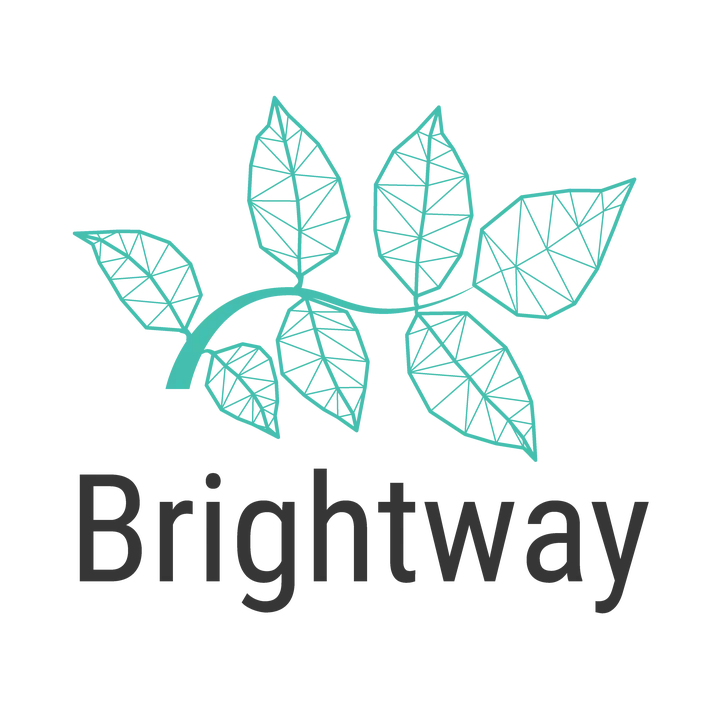
Introduction
Brightway2 is a Python library for Life Cycle Assessment (LCA). It is a powerful tool for performing LCA calculations, and it is widely used in the LCA community. Brightway2 is open-source and free to use, and it has a large and active community of users and developers.
In this post, we will show you how to get started with Brightway2. We will cover the basics of setting up a Brightway project, importing the ecoinvent database, and performing a simple LCA calculation.
Preqrequisites:
- Python
- Brightway2
- ecoinvent database
Install Python (windows, mac or linux)
Windows and Mac
- Download the latest version of Python from the official website: Python Downloads
- Run the installer and make sure to check the box that says “Add Python to PATH”
- Click “Install Now” and wait for the installation to complete
- Open a command prompt and type
python --version
to check that Python is installed correctly
Linux
Debian/Ubuntu
sudo apt update
sudo apt install python3
Fedora
sudo dnf install python3
CentOS/RHEL
sudo yum install python3
Install Anaconda (optional but we need it for this tutorial)
- Download the latest version of Anaconda from the official website: Anaconda Downloads
- Run the installer and follow the instructions
Install Brightway25
First create a new environment in Anaconda:
conda create -n bw python=3.11
Activate the environment:
conda activate bw
Install Brightway2:
pip install brightway25
From here on, you can use Brightway2 in your Python scripts or Jupyter notebooks. but you need to be sure to activate the environment before running your scripts or notebooks. Using an IDE like PyCharm or VSCode is also recommended.
Setting up a Brightway project with ecoinvent
Warning: This method is deprecated because
bw2setup
is deprecated. Use the newer method described below. Newer Method
import bw2data as bd
import bw2io as bi
The Classic way to set up a Brightway project with ecoinvent
def base_setup_with_ecoinvent(
project_name: str,
db_name: str,
db_loc: str,
reparametrize_lognormals: bool = True,
):
"""
This method sets up a Brightway project with the ecoinvent database.
Parameters
----------
db_name : str
The name of the database to import
db_loc : str
The location of the database to import (e.g. "C:/Users/.../ecoinvent 3.7.1_cutoff_ecoSpold02/datasets"))
reparametrize_lognormals : bool, optional
Whether or not to reparametrize lognormal distributions, by default True (recommended)
This sets the mean of the lognormal distribution to static value of the LCA calculation.
This is recommended because the default behavior of Brightway is to set the mean of the lognormal distribution to the value of the LCA calculation.
This can lead to problems when performing Monte Carlo LCA calculations, because the mean of the lognormal distribution will change with each iteration.
This can lead to a large number of iterations being required to reach convergence.
"""
# set the project, it will create a new project if it does not exist
bd.projects.set_current(project_name)
# imports biosphere flows, it will skip if already done
bi.bw2setup()
# get the path to the datasets folder
db_dir = db_loc
# give a name to the database
data_base_name = db_name
# check if the database is already in the project, and skip if yes
if data_base_name in bd.databases:
print("Database has already been imported")
else:
# import database
db = bi.SingleOutputEcospold2Importer(
db_dir,
data_base_name,
reparametrize_lognormals=reparametrize_lognormals,
)
db.apply_strategies()
db.statistics()
# db.drop_unlinked(True)
# writes database into sql
db.write_database()
print("Database has been imported")
How to use the function
base_setup_with_ecoinvent(
"project",
"ecoinvent 3.7.1_cutoff_ecoSpold02",
"C:/Users/.../ecoinvent 3.7.1_cutoff_ecoSpold02/datasets",
reparametrize_lognormals=True,
)
What does the function do?
This function sets up a Brightway project with the ecoinvent database. It imports the biosphere flows, and then imports the ecoinvent database. It also reparametrizes lognormal distributions, which is recommended because the default behavior of Brightway is to set the mean of the lognormal distribution to the value of the LCA calculation. This can lead to problems when performing Monte Carlo LCA calculations, because the mean of the lognormal distribution will change with each iteration. This can lead to a large number of iterations being required to reach convergence.
The newer approach to set up a Brightway project with ecoinvent
bd.projects.set_current("project")
bi.import_ecoinvent_release(version="3.9.1",
system_model="consequential", # or whatever system model you want
username="abc",
password="xyz")
What does the newer approach do?
It downloads the ecoinvent database from the internet and imports it into the Brightway project.
The fastest LCA of all time
import bw2data as bd
import bw2calc as bc
import bw2io as bi
import numpy as np
Activate the project
bd.projects.set_current("project")
bd.Database("ecoinvent 3.7.1_cutoff_ecoSpold02").random().lca(bd.methods.random()).score
Let’s break it down:
Pick a random activity
act = bd.Database("ecoinvent 3.7.1_cutoff_ecoSpold02").random()
Pick a random method
method = bd.methods.random()
Perform the LCA
act.lca(method).score
🎉 Yay! You’ve completed your first LCA with Brightway2! 🎉
Other useful functions
Get the list of methods
bd.methods
Get the list of databases
bd.databases
Get a list of projects
bd.projects
Choose and search a database
db = bd.Database("ecoinvent 3.7.1_cutoff_ecoSpold02")
db.search("electricity")
Choose and search a method
method = bd.Method(("IPCC 2013", "climate change", "GWP 100a"))
search for a method
method = [m for m in bd.methods if "GWP" in m[0]]
Get the activity as a dictionary
act.as_dict()
Backups and restores
backup the project directory
bi.backup.backup_project_directory('project')
This will create a zip file in the current directory with the name project.zip
which is stored based on your OS in the HOME directory.
restore the project directory
bi.backup.restore_project_directory('path')
What is Brightway?
refer to Brightway
What is ecoinvent?
refer to ecoinvent